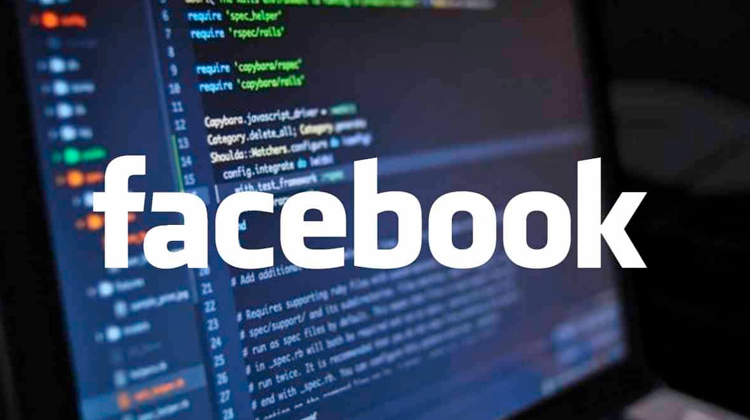
Connecting to the Facebook API is easy using this simple library. You'll need a little programming knowledge, but once you have it set up your app will be able retrieve all kinds of information from users and post updates on their behalf!
Note: Be sure to prepare the SDK on your development environment, if you have not done so already.
Full Code Example
// Begin loading the necessary files for the Facebook SDK.
require ( 'autoload.php' );
// Load the required PHP classes from the Facebook SDK.
use Facebook\FacebookSession;
use Facebook\FacebookRedirectLoginHelper;
use Facebook\FacebookRequest;
// Start the session
session_start();
// Initialize the Facebook app using the application ID and secret.
FacebookSession::setDefaultApplication( 'xxxxxxxxxxxxxx','xxxxxxxxxxxxxxxxxxxxxxxxxxx' );
// Define Facebook's login helper and redirect back to our page.
$helper = new FacebookRedirectLoginHelper( 'https://local.wordpress.dev/fb/index.php' );
// Check to ensure our session was started correctly and the access token exists.
if ( isset( $_SESSION ) && isset( $_SESSION['fb_token'] ) ) {
// Using the access token, create a new session.
$session = new FacebookSession( $_SESSION['fb_token'] );
// Determine if the defined session is indeed valid.
if ( !$session->validate() ) {
$session = null;
}
}
// Check if an active session exists.
if ( !isset( $session ) || $session === null ) {
// If no session exists, let's try to create one.
$session = $helper->getSessionFromRedirect();
}
// Make sure we have a session started.
if ( isset( $session ) ) {
// Save the session
$_SESSION['fb_token'] = $session->getToken();
// Create a new Facebook session using our token.
$session = new FacebookSession( $session->getToken() );
echo 'Connected to Facebook!';
} else {
// Show login url
echo 'Login';
}
Let's Look at This Code
Connecting to the Facebook SDK
First, we will need to connect to the Facebook SDK to load all of the required libraries. Thankfully, much of the work is already done for us with the autoload.php file that is included with the Facebook SDK. We simply need to include it and reference the required connection classes like so:
// Begin loading the necessary files for the Facebook SDK.
require ( 'autoload.php' );
// Load the required PHP classes from the Facebook SDK.
use Facebook\FacebookSession;
use Facebook\FacebookRedirectLoginHelper;
use Facebook\FacebookRequest;
If you noticed, we used the require() PHP function to include the autoload.php file. If you are using a PHP framework like Codeigniter or Laravel, you may need alter this code a bit.
Then, as our connection needs to load a session with Facebook, we have set our code to include the FacebookSession, FacebookRedirectLoginHelper, and FacebookRequest PHP classes to appropriately connect as well as request data from Facebook’s API.
Starting the session
Now that we have included the required libraries in our code, we need to open a new session. To do so, simply call the session_star() function:
// Start the session
session_start();
Initializing the connection to the Facebook API
So far, we have successfully started a session, but we’re still not yet connected to the Facebook API. To begin the connection, you will need to send your API key to Facebook’s API. The following lines will handle passing the API key on the Facebook:
// Initialize the Facebook app using the application ID and secret.
FacebookSession::setDefaultApplication( 'xxxxxxxxxxxxxx','xxxxxxxxxxxxxxxxxxxxxxxxxxx' );
Within this line, you will need to add your Application ID and Secret that is obtained from the Facebook Developers site. If you do not already have an Application ID, you may follow our article on obtaining a Facebook API key.
Now that the application is initialized and your credentials are verified with the Facebook API, a session needs to be created with Facebook. When creating sessions with the Facebook API, the user will be sent to Facebook to log in and authorize the application, then redirected back to your application. In the following lines, we are opening a session with Facebook and preparing to run requests to the Facebook API.
// Define Facebook's login helper and redirect back to our page.
$helper = new FacebookRedirectLoginHelper( 'https://local.wordpress.dev/fb/index.php' );
// Check to ensure our session was started correctly and the access token exists.
if ( isset( $_SESSION ) && isset( $_SESSION['fb_token'] ) ) {
// Using the access token, create a new session.
$session = new FacebookSession( $_SESSION['fb_token'] );
// Determine if the defined session is indeed valid.
if ( !$session->validate() ) {
$session = null;
}
}
// Check if an active session exists.
if ( !isset( $session ) || $session === null ) {
// If no session exists, let's try to create one.
$session = $helper->getSessionFromRedirect();
Prompt the user to login or display data
In the next lines, we then need to check to see if there is an active session, then display the content appopriately. If the user is not yet logged in and provided permissions to the application, the user will be prompted with a link to log in and will be returned to the page as per the FacebookRedirectLoginHelper URL. Once the user is returned, they will have a session active and will then be shown Connected to Facebook! in their browser.
// Make sure we have a session started.
if ( isset( $session ) ) {
// Save the session
$_SESSION['fb_token'] = $session->getToken();
// Create a new Facebook session using our token.
$session = new FacebookSession( $session->getToken() );
echo 'Connected to Facebook!';
} else {
// Show login url
echo 'Login';
}
Where do I go from here?
That's up to you! Go make a peanut butter & jelly sandwich (I prefer jam), take a walk, go watch a movie... just kidding! Now that you know how to easily connect to the Facebook API using the Facebook SDK for PHP, you can now make queries to Facebook to generate information on users.